10. pandas
和seaborn
: 統計圖表繪製#
xarray雖然可以輕易地開啟netCDF檔,繪製多種地圖,但目前沒有內建的統計圖表繪圖函數如盒鬚圖 (box plot)、散佈圖 (scatter plot) 等。seaborn
是強大的統計資料視覺化工具,可以利用簡明的語言和高階功能的引數,繪製專業又美觀的統計圖表。由於氣候資料的解讀很仰賴統計方法,因此學習利用如何將氣候統計的結果,送進 seaborn
的函數中繪圖,是很重要的。
seaborn
可以接受的資料格式主要為.csv資料檔,以及 pandas.DataFrame
,寫入資料時必須寫成 seaborn
能辨識之 「長表格 (long form)」 和 「寬表格 (wide form)」 ,有關表格的說明詳見seaborn
網頁的說明。本單元的重點在於如何建立正確的pandas.DataFrame
格式並且送進seaborn
的畫圖函數,有關繪圖的方法、引數等,官方教學已經有清楚的說明,此處不再一一介紹。
pandas
的資料架構#
按照資料的維度,pandas
的資料結構分為Series和DataFrame兩種。和xarray類似,pandas資料帶有網格資訊 (或稱為標籤 labels)。
Series#
Series是一維、標籤化的陣列,可以儲存多元的變數種類。而座標軸或標籤稱為index。建立Series的方法如下:
s = pd.Series(data, index=index)
只要給定資料和座標軸標籤,就可以建立Series。以下提供一個範例,更多詳細的用法請參考Pandas官網。
import numpy as np
import pandas as pd
s = pd.Series(np.random.randn(5), index=["a", "b", "c", "d", "e"])
s
a 2.517586
b -0.977689
c 1.620512
d -0.313335
e 0.274031
dtype: float64
DataFrame#
DataFrame就是二維標籤化的資料陣列,可以想像成一個Excel的活頁簿表格。建立的方法如下
s = pd.DataFrame(data, index=index, column=None)
index可以想像成列的標籤,column是欄的標籤。
d = np.random.randn(5,3)
df = pd.DataFrame(d, index=['a','b','c','d','e'], columns=['one','two','three'])
df
one | two | three | |
---|---|---|---|
a | 0.832259 | -0.751975 | -1.130962 |
b | 0.227706 | 1.563749 | 1.138527 |
c | 1.174121 | 1.192765 | -1.726206 |
d | -0.312869 | 0.576276 | 1.084590 |
e | 0.625784 | 1.227259 | 0.086139 |
也可以利用 字典 (Dictionary),而字典的key就會被當作欄的標籤。
df = pd.DataFrame(dict(bom=np.random.randn(10),
cma=np.random.randn(10),
ecmwf=np.random.randn(10),
ncep=np.random.randn(10)),
index=range(1998,2008)
)
df
bom | cma | ecmwf | ncep | |
---|---|---|---|---|
1998 | 2.552057 | -0.746450 | -0.158397 | 0.358142 |
1999 | -0.984721 | 0.380305 | -1.170051 | 1.557454 |
2000 | 1.280228 | -0.093588 | -0.689804 | -0.391513 |
2001 | 0.149104 | 0.812800 | 0.754814 | -0.600697 |
2002 | 0.203641 | 0.413762 | 0.260199 | -0.520028 |
2003 | 2.711357 | -1.626177 | 1.609361 | 0.233063 |
2004 | -0.285353 | -1.469338 | -1.061943 | -0.769027 |
2005 | -1.149144 | -0.189506 | -0.206343 | 1.781031 |
2006 | -1.802774 | -1.396945 | -1.178653 | -0.217372 |
2007 | -1.652627 | -0.120159 | 1.042927 | 0.163196 |
利用pandas
讀取.csv
檔案#
利用pandas.read_csv()
,就可以將.csv檔案轉換成 pandas.DataFrame
。
Example 1: sns_sample_s2s_pr_rmse.csv
檔案中有BoM、CMA的S2S模式在前15個預報時 (lead time),事後預報 (1998-2013) 某區域冬季季內高峰降雨事件的PR值的誤差 (PR_RMSE) 。(見Tsai et al. (2021, Atmosphere))
import pandas as pd
df = pd.read_csv("data/sns_sample_s2s_pr_rmse.csv")
df.head()
Models | Lead time (days) | Year | PR_RMSE | |
---|---|---|---|---|
0 | BoM | 1.0 | 1998.0 | 21.78 |
1 | BoM | 1.0 | 1999.0 | 36.98 |
2 | BoM | 1.0 | 2000.0 | 7.25 |
3 | BoM | 1.0 | 2001.0 | 13.18 |
4 | BoM | 1.0 | 2002.0 | 19.64 |
pandas.DataFrame
與seaborn
的Long Form繪圖#
只要將資料按照long form/wide form的需求排列好,就可以很輕易地將資料繪圖。以上的.csv檔案就是屬於Long form的形式。
Example 1: 將sns_sample_s2s_pr_rmse.csv
檔案繪圖,繪製x軸為預報時(Lead time),縱軸是預報PR_RMSE,利用盒鬚圖表示多年PR_RMSE的分布。
import matplotlib as mpl
from matplotlib import pyplot as plt
import seaborn as sns
mpl.rcParams['figure.dpi'] = 100
sns.set_theme(style="white", palette=None)
fig, ax = plt.subplots(figsize=(8,4))
bxplt = sns.boxplot(data=df,
x='Lead time (days)', y='PR_RMSE',
ax=ax,
hue='Models',
palette="Set3")
ax.set_ylabel("PR_RMSE")
plt.show()
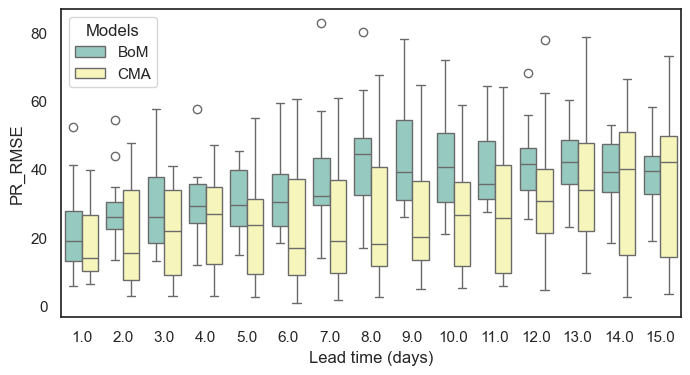
也可以用Facet Grid,將兩個模式分為兩張圖畫。用Facet Grid繪製盒鬚圖要用catplot()
這個函數。
sns.set_theme(style="white", palette=None)
bxplt = sns.catplot(data=df,
x='Lead time (days)', y='PR_RMSE',
kind='box', col='Models',
hue='Models',
palette="Set3")
ax.set_ylabel("PR_RMSE")
plt.show()
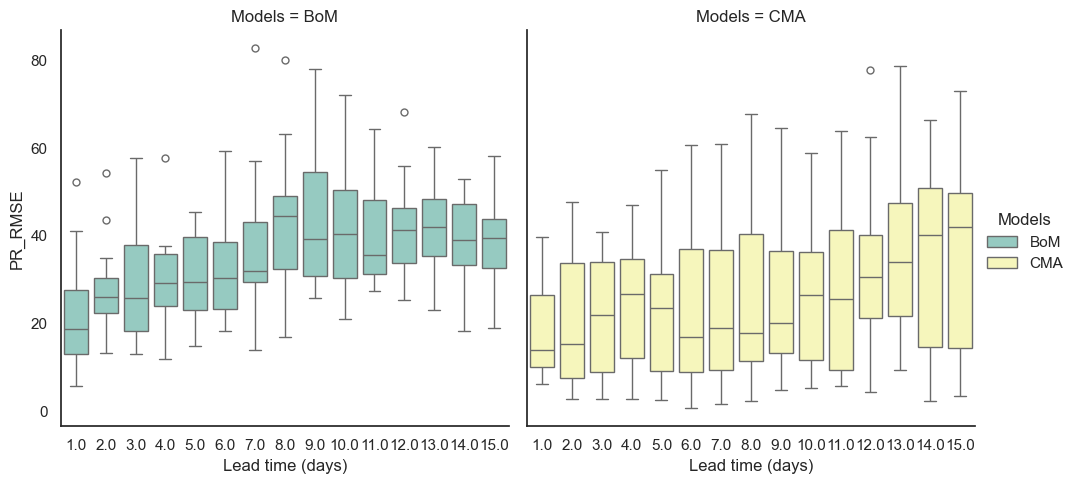
多層次標籤 (Multi-index) 的 DataFrame與Long Form繪圖#
Example 2: 分析S2S模式在15個預報時 (lead time, lt=15
) 以及11個系集成員 (ensemble members, ens=11
)在分為Hindcast、Forecast兩種cases的情形下,某變數value
的分佈情形。
由於value
分類的層次較多,所以必須用pandas.MultiIndex
建立起(lead_time, number, case)
的索引。
lt = 15
ens = 4
iterables = [range(1,lt+1), range(1,ens+1), ["Hindcast", "Forecast"]]
tuples = pd.MultiIndex.from_product(iterables, names=["lead_time", "number","case"])
# from_product 是將iterables中的標籤相乘,形成各lead time、number、case的組合。
data = pd.DataFrame(data={'value': np.random.randn(lt*ens*2)}, index=tuples)
# 先以亂數代表資料。資料取名為'value'。
data.head()
value | |||
---|---|---|---|
lead_time | number | case | |
1 | 1 | Hindcast | 0.770817 |
Forecast | -0.734005 | ||
2 | Hindcast | -0.823449 | |
Forecast | 0.657294 | ||
3 | Hindcast | -0.141362 |
我們發現表頭被分為兩行,這是因為在DataFrame
結構中, lead_time
、number
、case
稱為 Index,value
稱為 Column,如果直接放到seaborn
函數中,coulmns的名稱是無法使用的。
from matplotlib import pyplot as plt
sns.set_theme(style="white", palette=None)
bxplt = sns.catplot(data=data,
x='lead_time', y='value', kind='box',
hue='case', hue_order=['Hindcast','Forecast'],
palette=['white','silver'])
ax.set_ylabel("PR_RMSE")
plt.show()
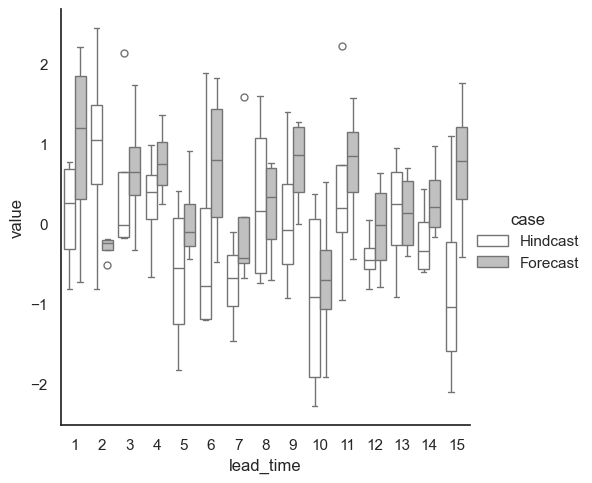
要讓這個DataFrame
變成seaborn
可讀取的long form格式,必須加上data.reset_index()
,就會轉變成理想中的DataFrame了。
data.reset_index()
lead_time | number | case | value | |
---|---|---|---|---|
0 | 1 | 1 | Hindcast | 0.770817 |
1 | 1 | 1 | Forecast | -0.734005 |
2 | 1 | 2 | Hindcast | -0.823449 |
3 | 1 | 2 | Forecast | 0.657294 |
4 | 1 | 3 | Hindcast | -0.141362 |
... | ... | ... | ... | ... |
115 | 15 | 2 | Forecast | 1.760044 |
116 | 15 | 3 | Hindcast | -0.671165 |
117 | 15 | 3 | Forecast | 1.018488 |
118 | 15 | 4 | Hindcast | -2.105161 |
119 | 15 | 4 | Forecast | 0.550923 |
120 rows × 4 columns
sns.set_theme(style="white", palette=None)
bxplt = sns.catplot(data=data.reset_index(),
x='lead_time', y='value', kind='box',
hue='case', hue_order=['Hindcast','Forecast'],
palette=['white','silver'],
aspect=1.5)
plt.show()
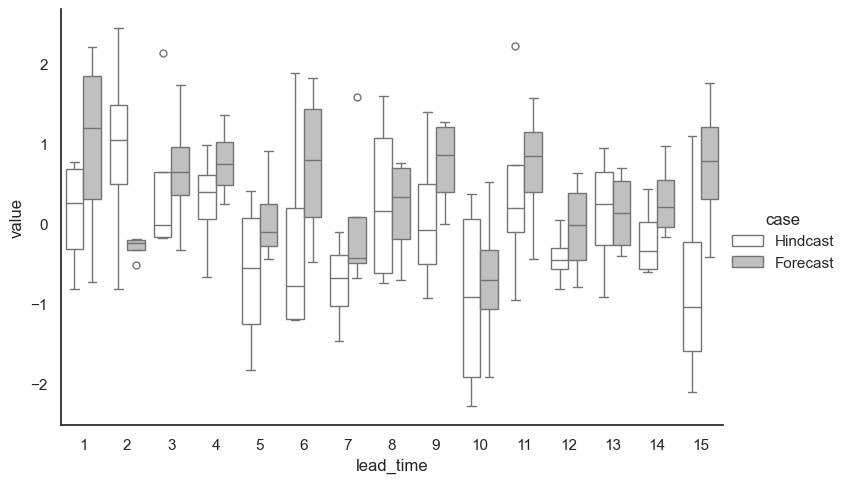
將xarray.DataArray
轉換至pandas.DataFrame
#
利用xarray.to_pandas
#
根據API reference的說明,轉換後的格式和給定DataArray的維度有關。
Convert this array into a pandas object with the same shape. The type of the returned object depends on the number of DataArray dimensions:
0D ->xarray.DataArray
1D ->pandas.Series
2D ->pandas.DataFrame
Only works for arrays with 2 or fewer dimensions.
範例:繪製散布圖 (scatter plot) 以及回歸分析#
Example 3: 將夏季(五至七月)候平均副高指標和長江流域(105.5˚-122˚E, 27˚-33.5˚N)降雨資料在散布圖上,並且計算回歸線。副高指數定義為
我們要了解降雨和副高兩個變量之間的關係,最常使用散佈圖來表示。兩個變量會用DataArray儲存,將兩個變量合併成一個Dataset,再轉換成pandas.DataFrame,就可以放到seaborn去作圖了。
Step 1: 讀取風場和降雨資料檔案。
import xarray as xr
pcpds = xr.open_dataset('data/cmorph_sample.nc')
pcp = (pcpds.sel(time=slice('1998-01-01','2018-12-31'),
lat=slice(27,33.5),
lon=slice(105.5,122)).cmorph)
pcp
/Users/waynetsai/.local/lib/python3.10/site-packages/ecmwflibs/__init__.py:83: UserWarning: dlopen(/Users/waynetsai/.local/lib/python3.10/site-packages/ecmwflibs/_ecmwflibs.cpython-310-darwin.so, 0x0002): tried: '/Users/waynetsai/.local/lib/python3.10/site-packages/ecmwflibs/_ecmwflibs.cpython-310-darwin.so' (mach-o file, but is an incompatible architecture (have 'x86_64', need 'arm64')), '/System/Volumes/Preboot/Cryptexes/OS/Users/waynetsai/.local/lib/python3.10/site-packages/ecmwflibs/_ecmwflibs.cpython-310-darwin.so' (no such file), '/Users/waynetsai/.local/lib/python3.10/site-packages/ecmwflibs/_ecmwflibs.cpython-310-darwin.so' (mach-o file, but is an incompatible architecture (have 'x86_64', need 'arm64'))
warnings.warn(str(e))
<xarray.DataArray 'cmorph' (time: 7670, lat: 26, lon: 66)> [13161720 values with dtype=float32] Coordinates: * time (time) datetime64[ns] 1998-01-01 1998-01-02 ... 2018-12-31 * lon (lon) float32 105.6 105.9 106.1 106.4 ... 121.1 121.4 121.6 121.9 * lat (lat) float32 27.12 27.38 27.62 27.88 ... 32.62 32.88 33.12 33.38 Attributes: standard_name: lwe_precipitation_rate long_name: precipitation units: mm/day ver_note: 1998-2020: V1,0; 2021: V0.x. comment: !!! CMORPH estimate is rainrate !!!
uds = xr.open_mfdataset( 'data/ncep_r2_uv850/u850.*.nc',
combine = "nested",
concat_dim='time',
parallel=True
)
u = uds.sel(time=slice('1998-01-01','2018-12-31'),
level=850,
lat=slice(30,15),
lon=slice(115,140)).uwnd
u
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 494 in H5O__attr_open_by_name(): can't locate attribute: '_QuantizeBitGroomNumberOfSignificantDigits'
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 494 in H5O__attr_open_by_name(): can't locate attribute: '_QuantizeGranularBitRoundNumberOfSignificantDigits'
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 494 in H5O__attr_open_by_name(): can't locate attribute: '_QuantizeBitRoundNumberOfSignificantBits'
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 494 in H5O__attr_open_by_name(): can't locate attribute: '_QuantizeBitGroomNumberOfSignificantDigits'
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 494 in H5O__attr_open_by_name(): can't locate attribute: '_QuantizeGranularBitRoundNumberOfSignificantDigits'
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 494 in H5O__attr_open_by_name(): can't locate attribute: '_QuantizeBitRoundNumberOfSignificantBits'
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 494 in H5O__attr_open_by_name(): can't locate attribute: '_QuantizeBitGroomNumberOfSignificantDigits'
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 494 in H5O__attr_open_by_name(): can't locate attribute: '_QuantizeGranularBitRoundNumberOfSignificantDigits'
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 494 in H5O__attr_open_by_name(): can't locate attribute: '_QuantizeBitRoundNumberOfSignificantBits'
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
HDF5-DIAG: Error detected in HDF5 (1.12.2) thread 0:
#000: H5A.c line 528 in H5Aopen_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#001: H5VLcallback.c line 1091 in H5VL_attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#002: H5VLcallback.c line 1058 in H5VL__attr_open(): attribute open failed
major: Virtual Object Layer
minor: Can't open object
#003: H5VLnative_attr.c line 130 in H5VL__native_attr_open(): can't open attribute
major: Attribute
minor: Can't open object
#004: H5Aint.c line 545 in H5A__open_by_name(): unable to load attribute info from object header
major: Attribute
minor: Unable to initialize object
#005: H5Oattribute.c line 476 in H5O__attr_open_by_name(): can't open attribute
major: Attribute
minor: Can't open object
#006: H5Adense.c line 394 in H5A__dense_open(): can't locate attribute in name index
major: Attribute
minor: Object not found
<xarray.DataArray 'uwnd' (time: 7670, lat: 7, lon: 11)> dask.array<getitem, shape=(7670, 7, 11), dtype=float32, chunksize=(366, 7, 11), chunktype=numpy.ndarray> Coordinates: * time (time) datetime64[ns] 1998-01-01 1998-01-02 ... 2018-12-31 * lon (lon) float32 115.0 117.5 120.0 122.5 ... 132.5 135.0 137.5 140.0 * lat (lat) float32 30.0 27.5 25.0 22.5 20.0 17.5 15.0 level float32 850.0 Attributes: (12/14) standard_name: eastward_wind long_name: Daily U-wind on Pressure Levels units: m/s unpacked_valid_range: [-140. 175.] actual_range: [-78.96 110.35] precision: 2 ... ... var_desc: u-wind dataset: NCEP/DOE AMIP-II Reanalysis (Reanalysis-2) Daily A... level_desc: Pressure Levels statistic: Mean parent_stat: Individual Obs cell_methods: time: mean (of 4 6-hourly values in one day)
Step 2: 計算候降雨區域平均和副高指標,並且取出所需要的季節。
pcpts = (pcp.mean(axis=(1,2))
.sel(time=~((pcp.time.dt.month == 2) & (pcp.time.dt.day == 29)))
)
pcp_ptd = pcpts.coarsen(time=5,side='left', coord_func={"time": "min"}).mean() # 計算pentad mean
pcp_ptd_mjj = pcp_ptd.sel(time=(pcp_ptd.time.dt.month.isin([5,6,7])))
pcp_ptd_mjj
<xarray.DataArray 'cmorph' (time: 399)> array([ 4.8970165 , 7.9518876 , 6.319289 , 2.4725406 , 5.27035 , 2.170851 , 4.783776 , 3.937529 , 11.973356 , 10.144266 , 11.061295 , 13.090139 , 9.517157 , 2.0094523 , 3.4387412 , 6.7226806 , 11.502437 , 10.5547905 , 9.479826 , 2.9567833 , 3.5055594 , 4.1681933 , 6.725932 , 11.227705 , 2.9874592 , 2.4019814 , 4.487879 , 2.9615617 , 9.328345 , 8.361376 , 17.8 , 5.0629954 , 9.045652 , 4.8956294 , 9.328997 , 3.7565734 , 3.8314571 , 4.111655 , 0.3021329 , 3.6132984 , 3.5981002 , 2.3257692 , 7.2976217 , 5.5318065 , 13.691736 , 7.9762588 , 4.2428904 , 3.8893006 , 13.693474 , 6.9901514 , 9.405932 , 4.689091 , 6.358776 , 3.2129135 , 2.252133 , 7.383217 , 2.8664687 , 4.964475 , 4.936305 , 1.1263635 , 2.2311187 , 2.2804198 , 4.0579023 , 8.117238 , 6.8227034 , 5.0488696 , 8.666551 , 5.666422 , 4.5268536 , 3.3424478 , 3.6482053 , 6.963858 , 1.558951 , 3.8080535 , 2.550478 , 5.6249647 , 9.637308 , 6.783858 , 12.585258 , 4.844767 , 1.3790094 , 4.6789045 , 1.5510608 , 7.1460257 , 5.1195927 , 7.1560845 , 12.34908 , 12.694429 , 3.5173545 , 2.4259324 , 0.28142193, 9.145932 , 12.452902 , 4.6736712 , 5.1581006 , 6.8205705 , 4.7389283 , 9.617587 , 3.4697907 , 3.9260025 , ... 5.9538927 , 5.01324 , 0.9436598 , 4.4753027 , 6.041911 , 6.934068 , 5.07711 , 4.8284035 , 8.588112 , 1.5448952 , 10.228043 , 1.7740676 , 1.9260607 , 7.228357 , 8.549138 , 6.3187065 , 12.640233 , 1.4202797 , 11.316084 , 7.279161 , 4.142191 , 3.5260372 , 2.4751165 , 4.1878905 , 7.2561064 , 11.466655 , 4.0412 , 1.946387 , 7.6451874 , 10.063392 , 6.7887535 , 6.0969815 , 12.194288 , 8.405164 , 10.297377 , 7.8729143 , 2.699021 , 4.153112 , 5.390607 , 10.084627 , 3.1376457 , 2.0847204 , 9.51324 , 11.786633 , 3.1671562 , 5.3714223 , 4.1579022 , 5.4215965 , 14.319791 , 3.3686714 , 4.624697 , 13.882413 , 12.359255 , 10.334114 , 21.119303 , 5.0222025 , 7.4891496 , 11.105817 , 0.966352 , 3.1370628 , 6.3480887 , 4.5376463 , 4.9669466 , 7.596236 , 1.2960141 , 4.0143824 , 0.98304194, 9.380851 , 8.573579 , 11.14049 , 4.2023306 , 13.881643 , 9.043998 , 9.088369 , 9.648952 , 4.0862937 , 3.827599 , 0.41491842, 1.7904428 , 4.379033 , 6.227797 , 4.3578324 , 1.9046853 , 12.030944 , 7.8016205 , 5.260373 , 2.5838928 , 3.4374127 , 2.6103146 , 6.182331 , 4.548438 , 7.4217596 , 12.894266 , 7.690734 , 2.6264687 , 0.7665617 , 2.525 , 7.5979834 , 5.8932753 ], dtype=float32) Coordinates: * time (time) datetime64[ns] 1998-05-01 1998-05-06 ... 2018-07-30
ushear = ( u.sel(lat=slice(30,28)).mean(axis=(1,2)) -
u.sel(lat=slice(17,15)).mean(axis=(1,2)) )
ushear_ts = ushear.sel(time=~((ushear.time.dt.month == 2) & (ushear.time.dt.day == 29)))
us_ptd = ushear_ts.coarsen(time=5,side='left', coord_func={"time": "min"}).mean() # 計算pentad mean
us_ptd_mjj = us_ptd.sel(time=(us_ptd.time.dt.month.isin([5,6,7])))
us_ptd_mjj
<xarray.DataArray 'uwnd' (time: 399)> dask.array<getitem, shape=(399,), dtype=float32, chunksize=(19,), chunktype=numpy.ndarray> Coordinates: * time (time) datetime64[ns] 1998-05-01 1998-05-06 ... 2018-07-30 level float32 850.0
Step 3: 轉換成DataFrame的Long Form形式,並送入seaborn
繪圖函數繪圖。繪製散布圖以及對應的迴歸線,使用seaborn.regplot
這個函數。記得將dataset轉換成Long Form的DataFrame時,要加上reset_index()
。
scatter_df = (xr.merge([pcp_ptd_mjj.rename('pcp'), us_ptd_mjj.rename('ushear')])
.to_dataframe()
.reset_index())
scatter_df
time | pcp | level | ushear | |
---|---|---|---|---|
0 | 1998-05-01 | 4.897017 | 850.0 | 10.862909 |
1 | 1998-05-06 | 7.951888 | 850.0 | 11.484545 |
2 | 1998-05-11 | 6.319289 | 850.0 | 12.718000 |
3 | 1998-05-16 | 2.472541 | 850.0 | 7.670909 |
4 | 1998-05-21 | 5.270350 | 850.0 | -9.089272 |
... | ... | ... | ... | ... |
394 | 2018-07-10 | 2.626469 | 850.0 | -5.535454 |
395 | 2018-07-15 | 0.766562 | 850.0 | -14.066727 |
396 | 2018-07-20 | 2.525000 | 850.0 | -11.340726 |
397 | 2018-07-25 | 7.597983 | 850.0 | -6.049819 |
398 | 2018-07-30 | 5.893275 | 850.0 | -6.609454 |
399 rows × 4 columns
from scipy import stats
def corr(x, y):
return stats.pearsonr(x, y)[0], stats.pearsonr(x, y)[1]
# 計算相關係數和統計顯著性。
r, p = corr(us_ptd_mjj.values, pcp_ptd_mjj.values)
fig, ax = plt.subplots(figsize=(8,8))
sns.set_theme()
plot = sns.regplot(x="ushear", y="pcp",
data=scatter_df,
ci=95, ax=ax) # ci是信心水準
ax.set_title(f'$R=$ {r:5.3f}, $p=$ {p:8.2e}', loc='right' )
Text(1.0, 1.0, '$R=$ 0.394, $p=$ 3.07e-16')
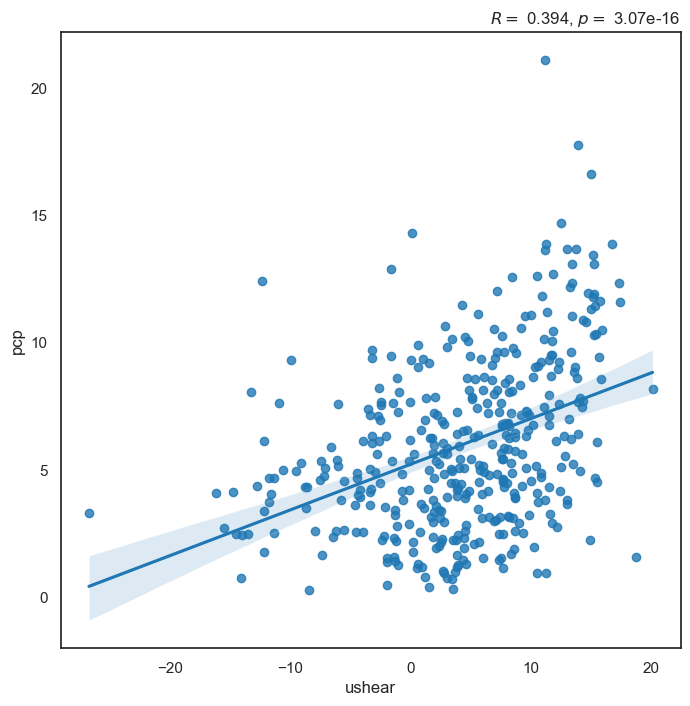
Wide Form的Seaborn製圖#
Example 4: 繪製台灣-北南海 (18˚-24˚N, 116˚-126˚E) 區域平均 1998-2020 各年四至十一月逐候 (pentad) 累積降雨百分等級 (PR) 的Heat Map。
# 台灣
lats = 18
latn = 24
lon1 = 116
lon2 = 126
pcp = pcpds.sel(time=slice('1998-01-01','2020-12-31'),
lat=slice(lats,latn),
lon=slice(lon1,lon2)).cmorph
pcp_ptd_ts = (pcp.mean(axis=(1,2))
.sel(time=~((pcp.time.dt.month == 2) & (pcp.time.dt.day == 29)))
.coarsen(time=5,side='left', coord_func={"time": "min"})
.sum())
pcp_season = pcp_ptd_ts.sel(time=(pcp_ptd_ts.time.dt.month.isin([4,5,6,7,8,9,10,11])))
# 建立降雨的氣候基期
pcp_rank = pcp_season.rank(dim='time',pct=True) * 100. # 利用DataArray.rank計算排名,pct=True可將排名百分化
pcp_rank_da = xr.DataArray(data=pcp_rank.values.reshape(23,49), # reshape將矩陣重塑成(year, pentad)的形狀
dims=["year", "pentad"],
coords=dict(
year = range(1998,2021,1),
pentad = range(19,68,1),
),
name='precip')
pcp_rank_da
<xarray.DataArray 'precip' (year: 23, pentad: 49)> array([[2.40461402e+01, 7.98580302e-01, 7.22271517e+01, ..., 6.67258208e+01, 8.33185448e+01, 3.62910382e+01], [3.99290151e+01, 1.87222715e+01, 6.03371783e+01, ..., 2.72404614e+01, 1.30434783e+01, 7.00976043e+01], [4.31233363e+01, 3.47826087e+01, 4.62289264e+01, ..., 6.34427684e+01, 4.42768412e+01, 6.29991127e+00], ..., [8.87311446e-02, 3.44276841e+01, 4.09937888e+01, ..., 1.56166815e+01, 5.49245785e+01, 3.02573203e+01], [4.30346051e+01, 2.66193434e-01, 5.42147294e+01, ..., 9.13930790e+01, 3.16770186e+01, 1.20674357e+01], [4.27684117e+01, 5.04880213e+01, 2.71517303e+01, ..., 1.48181012e+01, 1.27772848e+01, 4.57852706e+01]]) Coordinates: * year (year) int64 1998 1999 2000 2001 2002 ... 2016 2017 2018 2019 2020 * pentad (pentad) int64 19 20 21 22 23 24 25 26 ... 60 61 62 63 64 65 66 67
pcp_rank_df = pcp_rank_da.to_pandas()
pcp_rank_df
pentad | 19 | 20 | 21 | 22 | 23 | 24 | 25 | 26 | 27 | 28 | ... | 58 | 59 | 60 | 61 | 62 | 63 | 64 | 65 | 66 | 67 |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
year | |||||||||||||||||||||
1998 | 24.046140 | 0.798580 | 72.227152 | 77.107365 | 32.475599 | 68.411713 | 51.197870 | 26.086957 | 45.519077 | 86.867791 | ... | 94.942325 | 43.300799 | 98.225377 | 15.527950 | 66.104703 | 52.440106 | 20.585626 | 66.725821 | 83.318545 | 36.291038 |
1999 | 39.929015 | 18.722272 | 60.337178 | 5.501331 | 7.897072 | 81.366460 | 70.275067 | 76.220053 | 55.723159 | 49.778172 | ... | 85.891748 | 64.951198 | 10.559006 | 24.312334 | 28.748891 | 13.487134 | 12.156167 | 27.240461 | 13.043478 | 70.097604 |
2000 | 43.123336 | 34.782609 | 46.228926 | 26.974268 | 34.605146 | 58.207631 | 39.219166 | 4.081633 | 70.541260 | 84.117125 | ... | 69.565217 | 48.890861 | 25.554570 | 96.628217 | 88.553682 | 65.838509 | 65.306122 | 63.442768 | 44.276841 | 6.299911 |
2001 | 39.751553 | 37.267081 | 37.799468 | 40.195209 | 61.579414 | 22.448980 | 37.089618 | 58.385093 | 92.280390 | 77.639752 | ... | 55.989352 | 5.944987 | 1.863354 | 26.619343 | 15.882875 | 57.409051 | 66.637090 | 6.654836 | 4.170364 | 2.040816 |
2002 | 4.259095 | 3.637977 | 21.916593 | 6.743567 | 3.992902 | 4.702751 | 0.177462 | 3.460515 | 33.895297 | 79.858030 | ... | 11.002662 | 49.423248 | 43.212067 | 49.068323 | 34.072760 | 50.399290 | 17.125111 | 62.999113 | 26.796806 | 25.820763 |
2003 | 48.003549 | 56.255546 | 20.496894 | 31.322094 | 78.615794 | 7.808341 | 37.533274 | 6.122449 | 11.268855 | 47.914818 | ... | 44.454303 | 11.712511 | 2.750665 | 61.490683 | 57.231588 | 27.861579 | 64.596273 | 50.842946 | 46.140195 | 8.340728 |
2004 | 44.099379 | 18.367347 | 21.118012 | 17.746229 | 5.678793 | 8.518190 | 9.671695 | 30.434783 | 19.698314 | 77.994676 | ... | 15.705413 | 38.509317 | 62.910382 | 1.685892 | 3.194321 | 13.753327 | 19.343390 | 42.945874 | 56.965395 | 28.926353 |
2005 | 7.187223 | 1.419698 | 26.264419 | 8.074534 | 18.988465 | 11.801242 | 17.480035 | 86.956522 | 61.668146 | 27.595386 | ... | 13.664596 | 9.405501 | 29.281278 | 56.166815 | 0.976043 | 42.502218 | 50.044366 | 66.015972 | 33.007986 | 50.931677 |
2006 | 2.218279 | 15.350488 | 46.406389 | 34.960071 | 19.254658 | 49.334516 | 27.950311 | 3.105590 | 69.476486 | 81.543922 | ... | 5.590062 | 11.446318 | 33.984028 | 87.133984 | 72.848270 | 29.547471 | 35.492458 | 54.303461 | 21.827862 | 33.096717 |
2007 | 51.020408 | 33.185448 | 5.767524 | 39.396628 | 27.772848 | 13.398403 | 32.386868 | 52.706300 | 30.967169 | 74.090506 | ... | 30.612245 | 12.954747 | 20.053239 | 54.835847 | 91.481810 | 75.421473 | 7.364685 | 25.199645 | 87.488909 | 43.833185 |
2008 | 12.333629 | 3.904170 | 12.599823 | 48.269743 | 51.641526 | 43.389530 | 46.850044 | 30.079858 | 56.433008 | 71.960958 | ... | 72.315883 | 7.630878 | 14.374445 | 10.825200 | 19.964508 | 93.611358 | 32.209406 | 54.037267 | 62.732919 | 47.204969 |
2009 | 30.700976 | 38.952972 | 50.754215 | 81.721384 | 90.683230 | 40.550133 | 12.688554 | 55.013310 | 0.532387 | 20.763088 | ... | 59.982254 | 72.049689 | 70.629991 | 33.362910 | 60.425909 | 4.525288 | 48.624667 | 46.761313 | 22.626442 | 19.432121 |
2010 | 14.640639 | 35.314996 | 32.564330 | 24.134871 | 33.629104 | 76.841171 | 64.063886 | 21.472937 | 24.755989 | 9.849157 | ... | 67.701863 | 96.716948 | 63.975155 | 23.336291 | 78.083407 | 72.759539 | 80.745342 | 36.645963 | 41.171251 | 10.204082 |
2011 | 10.647737 | 2.484472 | 1.153505 | 58.473824 | 18.101154 | 18.012422 | 10.026619 | 73.469388 | 26.530612 | 86.335404 | ... | 87.577640 | 44.986690 | 29.458740 | 33.451642 | 67.435670 | 94.676131 | 80.035492 | 65.572316 | 50.221828 | 68.677906 |
2012 | 31.588287 | 36.557232 | 1.597161 | 56.699201 | 22.981366 | 64.507542 | 37.976930 | 19.875776 | 54.392192 | 97.249335 | ... | 13.575865 | 3.726708 | 37.444543 | 59.804791 | 69.653949 | 12.244898 | 52.085182 | 59.094942 | 35.758651 | 39.041704 |
2013 | 59.538598 | 64.685004 | 31.410825 | 24.578527 | 29.724933 | 57.941437 | 75.332742 | 28.305235 | 66.193434 | 72.138421 | ... | 39.840284 | 14.285714 | 23.425022 | 76.929902 | 48.447205 | 35.048802 | 42.857143 | 23.070098 | 41.259982 | 42.058563 |
2014 | 49.955634 | 24.489796 | 7.275954 | 0.709849 | 10.292813 | 30.346051 | 64.862467 | 73.824312 | 36.202307 | 37.000887 | ... | 7.985803 | 35.403727 | 34.693878 | 4.436557 | 16.592724 | 9.937888 | 66.903283 | 51.286602 | 16.503993 | 41.881100 |
2015 | 25.643301 | 42.324756 | 39.574091 | 34.871340 | 41.703638 | 16.149068 | 10.115350 | 59.449867 | 38.065661 | 52.883762 | ... | 52.173913 | 85.714286 | 27.417924 | 17.213842 | 54.569654 | 31.144632 | 20.408163 | 28.482697 | 41.614907 | 25.377107 |
2016 | 0.621118 | 40.638864 | 67.169476 | 9.228039 | 29.192547 | 37.355812 | 13.220941 | 20.230701 | 50.310559 | 58.651287 | ... | 69.387755 | 80.390417 | 8.163265 | 46.938776 | 14.729370 | 25.022183 | 16.770186 | 45.430346 | 74.445430 | 81.810115 |
2017 | 19.165927 | 1.952085 | 47.116238 | 26.885537 | 59.361136 | 36.379769 | 10.913931 | 11.623780 | 50.133097 | 95.563443 | ... | 90.417036 | 24.667258 | 11.978705 | 28.837622 | 86.069210 | 64.330080 | 47.648625 | 48.802130 | 46.583851 | 53.149956 |
2018 | 0.088731 | 34.427684 | 40.993789 | 18.456078 | 37.178350 | 6.921029 | 11.091393 | 65.394854 | 4.791482 | 1.064774 | ... | 46.495120 | 28.393966 | 11.357587 | 91.215617 | 23.158829 | 1.774623 | 16.681455 | 15.616681 | 54.924579 | 30.257320 |
2019 | 43.034605 | 0.266193 | 54.214729 | 69.742680 | 8.606921 | 29.015084 | 78.172138 | 75.865129 | 47.293700 | 46.051464 | ... | 24.223602 | 14.995563 | 19.520852 | 37.888199 | 88.819876 | 47.826087 | 38.243123 | 91.393079 | 31.677019 | 12.067436 |
2020 | 42.768412 | 50.488021 | 27.151730 | 1.330967 | 47.471162 | 29.103815 | 3.549246 | 4.880213 | 42.590949 | 89.263531 | ... | 45.607808 | 84.472050 | 79.680568 | 53.238687 | 74.001775 | 76.131322 | 77.817214 | 14.818101 | 12.777285 | 45.785271 |
23 rows × 49 columns
以上的DataFrame就是一個Wide Form的形式。
Long Form的表格,索引標籤都只存在index裡;Wide Form的表格,則是由Column和Index共同組成資料的內容,並且以2維的形式呈現。
# Plot
fig, ax = plt.subplots(figsize=(12,8))
sns.set_theme()
ax = sns.heatmap(pcp_rank_df,
cmap='jet_r',
square=True,
vmin=1,vmax=100,
cbar_kws={"shrink": 0.55, 'extend':'neither'},
xticklabels=2)
plt.xlabel("Pentad")
plt.ylabel("Years")
ax.set_title("Taiwan-Northern SCS, April to November",loc='left')
plt.savefig("pcp_pr_heatmap_obs_chn.png",orientation='portrait',dpi=300)

Wide/Long form互相轉換#
利用pandas.DataFrame.unstack
:
pcp_rank_long = pcp_rank_df.unstack().reset_index(name='PR')
pcp_rank_long
pentad | year | PR | |
---|---|---|---|
0 | 19 | 1998 | 24.046140 |
1 | 19 | 1999 | 39.929015 |
2 | 19 | 2000 | 43.123336 |
3 | 19 | 2001 | 39.751553 |
4 | 19 | 2002 | 4.259095 |
... | ... | ... | ... |
1122 | 67 | 2016 | 81.810115 |
1123 | 67 | 2017 | 53.149956 |
1124 | 67 | 2018 | 30.257320 |
1125 | 67 | 2019 | 12.067436 |
1126 | 67 | 2020 | 45.785271 |
1127 rows × 3 columns